Extra bytes in received message
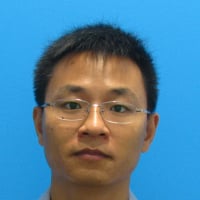
I use a simple JCSMP consumer to consume data from a broker, here is the code:
When messages are received, there are always some extra bytes at the beginning of the buffer. for example, when I publish a TextMessage with content "Hello World!" the received data is
The first 2 bytes are extra.
When I use Postman to post a message with body "123", the received data is:
There are 4 extra bytes at the begining of the buffer.
What is the cause of the extra bytes?
Seems the same problem has been asked in https://stackoverflow.com/questions/48647787/invald-characters-at-start-of-message-from-solace-mq
Best Answer
-
Your publisher is publishing as Text messages, which are a Structured Data Type (SDT) message, but trying to read as binary Bytes message. A TextMessage is a special type of SdtStream message, that only holds a single data element, the string payload. The first couple bytes you're seeing (could be 2-6 bytes) are the length of the encoded String.
Generally, you do something like:
if (msg instanceof TextMessage) { TextMessage tMsg = (TextMessage)msg; String payload = tMsg.getText(); } else if (msg instanceof BytesMessage) { BytesMessage bMsg = (BytesMessage)msg; // if you know the payload is a String... String payload = new String(bMsg.getData()); } else ...
Something like that! Check what type the received message is and cast to that and work with that, rather than the supertype. Note: I'm just writing that out free-hand (might not be exact working code). But should point you in the right direction.
1
Answers
-
Your publisher is publishing as Text messages, which are a Structured Data Type (SDT) message, but trying to read as binary Bytes message. A TextMessage is a special type of SdtStream message, that only holds a single data element, the string payload. The first couple bytes you're seeing (could be 2-6 bytes) are the length of the encoded String.
Generally, you do something like:
if (msg instanceof TextMessage) { TextMessage tMsg = (TextMessage)msg; String payload = tMsg.getText(); } else if (msg instanceof BytesMessage) { BytesMessage bMsg = (BytesMessage)msg; // if you know the payload is a String... String payload = new String(bMsg.getData()); } else ...
Something like that! Check what type the received message is and cast to that and work with that, rather than the supertype. Note: I'm just writing that out free-hand (might not be exact working code). But should point you in the right direction.
1 -
What API is the publisher of these bytes messages? Can we see some info on that? To that end, which of the "if"s is the message being processed by? The XMLContent or the Binary Attachment?
0 -
I think @Aaron is likely on the right track here. Are you setting a HTTP Content-Type in postman?
You can see how the content-types map to Solace message type in the "HTTP Content-Type Mapping to Solace Message Types" section here: https://docs.solace.com/API/RESTMessagingPrtl/Solace-REST-Message-Encoding.htm#_Ref393979978
1