🎄 Happy Holidays! 🥳
Most of Solace is closed December 24–January 1 so our employees can spend time with their families. We will re-open Thursday, January 2, 2024. Please expect slower response times during this period and open a support ticket for anything needing immediate assistance.
Happy Holidays!
Please note: most of Solace is closed December 25–January 2, and will re-open Tuesday, January 3, 2023.
Quick-and-dirty REST server for RDP testing
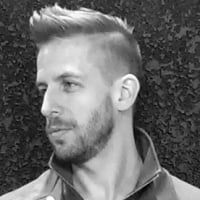
Hi there! I thought I'd share a (possibly!) useful snippet of code that you can use to receive REST requests, and respond with a canned body. It's in Node. I don't know Node, so apologies if it's not glamourous code. I was inspired by the "Simple REST Consumer" on this tutorial: https://solace.com/samples/solace-samples-rest-messaging/publish-subscribe/
Update the variable RC_HOST
at the top of the file with your machine's IP address. Don't use localhost
, use the actual IP address. Note, if using WSL2, you'll need to use the "internal" address of the virtual machine. On my Windows machine, I run this from the Ubuntu shell, so my IP address looks like 172.28.123.115
.
And if running the PubSub+ broker locally, inside your RDP Rest Consumer, you can't use "localhost" or "127.0.0.1" when testing with an RDP, because the Solace broker has it's own networking setup. You'll have to specify your actual IP address, or whatever you started the server with.
Anyhow, copy this into a file, and then simply run node NodeServer.js
% cat NodeServer.js var http = require('http'); var RC_PORT = 9090; //var RC_HOST = '127.0.0.1'; // only works from browser, not RDP //var RC_HOST = '10.1.1.245'; // home var RC_HOST = '192.168.42.194'; // office LAN http.createServer(function (req, res) { let date_ob = new Date(); var dateStr = date_ob.getHours()+':'+date_ob.getMinutes()+':'+date_ob.getSeconds(); //console.log(req); // all the details of this request console.log('Received message: ' + req.method + " " + req.url + ' at ' + dateStr); Object.keys(req.headers).forEach(function(key) { //if (!key.startsWith('solace')) return; var val = req.headers[key]; console.log(' HEAD:' + key + ' = ' + val); }); let body = []; req.on('data', (chunk) => { body.push(chunk); }).on('end', () => { body = Buffer.concat(body).toString(); // at this point, `body` has the entire request body stored in it as a string console.log(' BODY: '+ body); }); // RESPONSE TIME! //res.writeHead(200); // bytes message res.writeHead(200, { 'Content-Type': 'text/plain' }); // text message res.write("Hello world from Aaron's test HTTP server!"); res.end(); //console.log(res); }).listen(RC_PORT,RC_HOST); // good to go! console.log('Server running at http://'+RC_HOST+':'+RC_PORT+'/');
When you send a request, it will dump stuff out to the console. Here's a quick test from Chrome, loading http://192.168.42.194:9090/hello/world
:
Server running at http://192.168.42.194:9090/ Received message: GET /hello/world at 15:0:48 HEAD:host = 192.168.42.194:9090 HEAD:connection = keep-alive HEAD:upgrade-insecure-requests = 1 HEAD:user-agent = Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36 HEAD:accept = text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9 HEAD:accept-encoding = gzip, deflate HEAD:accept-language = en-GB,en-US;q=0.9,en;q=0.8 BODY:
Have fun playing with RDPs and Solace+REST!
Comments
-
Bump! I just found out that Solace's beloved command line test tool
SdkPerf
has a REST mode, and can act as a webhook endpoint, echoing incoming REST requests from RDPs, just like my above Node webserver.- Head to https://solace.com/downloads
- Click the box that says "Other"
- Fill in your details
- Download the REST version:
Then from the command line, pretty much all you need is to tell it which port to bind to using the
-spl
parameter:./sdkperf_rest.sh -spl=12345 -md -q
Then if I do the same test as above, just using Chrome to verify:
http://localhost:12345/hello/world
:^^^^^^^^^^^^^^^^^^ Start Message ^^^^^^^^^^^^^^^^^^^^^^^^^^^ Post-Request-URI : %2Fhello%2Fworld DeliveryMode : PERSISTENT Headers Cookie : _ga=GA1.1.567890820.1615794484; fs_uid=rs.fullstory.com#13AXQ4#6077823464579072:4684159190376448#0ef8131a#/1656476679; ajs_user_id=d071d4c2-e717-41ac-b344-3ccd9f8d10cd; ajs_anonymous_id=632237e8-ab12-4e22-9036-37b493ca8bc6; TSID=8dfa125aa9f4a4b9 Accept : text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9 Connection : keep-alive User-Agent : Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/94.0.4606.71 Safari/537.36 Sec-Fetch-Site : none Sec-Fetch-Dest : document Host : localhost:12345 Accept-Encoding : gzip, deflate, br Sec-Fetch-Mode : navigate sec-ch-ua : "Chromium";v="94", "Google Chrome";v="94", ";Not A Brand";v="99" sec-ch-ua-mobile : ?0 Upgrade-Insecure-Requests : 1 sec-ch-ua-platform : "Windows" Sec-Fetch-User : ?1 Accept-Language : en-GB,en-US;q=0.9,en;q=0.8 Parms NanoHttpd.QUERY_STRING : /hello/world ^^^^^^^^^^^^^^^^^^ End Message ^^^^^^^^^^^^^^^^^^^^^^^^^^^
Awesome! Remember, you need to configure your RDP REST Consumer remote host with your host computer's actual IP address, not just "localhost" or 127.0.0.1.
4